Modularization Techniques in SAP ABAP
Modularization
Techniques in SAP ABAP
Objective:
We can use few techniquesf, if the program contains the same or similar blocks
of statements or
it is required to process the same function several times
these techniques are Modularization Techniques.
Modularization
Modularization is nothing but breaking down and organizing
program's code
into a modular structure, rather than dealing with the
program as a single unit.
Where to we use Modularization Techniques
Output:
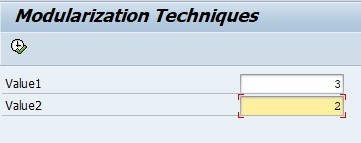
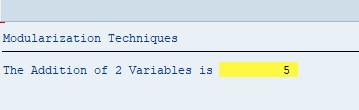
Save-> Check-> Activate and Observe the Output.
-Function modules are stored in a Central Library. (i.e., Function Group)
-Function modules are having reusable logic/code
Where to use the function modules?
Pre-requisites to create a Function Module
If you get 3 requirements related to 'Employee' like mentioned below:
Note:




Execute Transaction SE38
In side ABAP Code (which can be written by using
Transactions SE38/SE37/SE24/SE80).
Use of modularization
1) Improves readability
-> Easy to read/Analysis of the code.
2) Re-usability
-> can be used in other programs as well.
3) Encapsulation of data
-> Bundling/making a specific code in a single unit.
4) Reduces code redundancies-> to avoid duplication of
code.
Modularization Techniques (Reusable
Techniques):
1.Macros
2.Includes
3.Subroutines
4.Function Modules
5.Methods (OOABAP related)
1.Macros
Macros are another way
to modularize code, but they’re considered obsolete technology and shouldn’t be used.
Macros are callable modules of program code.
Macros are difficult to debug, but can be used to run a
statement more than once in a code.
The Variables/values
passed during the macro call are received into &1 &2 …..&9 in a
macro definition.
Syntax for
defining and calling Macros:
Define Macros:
*& Syntax
DEFINE <Macro Name>.
<statements> using
&1 & 2 &3...…&9.
ENDDEFINE.
Calling Macros
*&--Syntax
<Macro name> [
<P1> <p2> …. <p9>].
Example:
Write a program to perform arithmetic operations of 2 inputs
by using macros.
Code:
REPORT ZMODULARIZATION_TECHNIQUES1.
DATA output TYPE i.
PARAMETERS: p_input1 TYPE i,
p_input2 TYPE i.
*& Define a macro
DEFINE operations.
output = &1
&2 &3.
WRITE:/ 'The result
of &1 &2 &3 is', output.
END-OF-DEFINITION.
*& Call a macro
operations p_input1 + p_input2.
operations p_input1 * p_input2.
operations p_input1 - p_input2.
2.Include Programs
(It is also one type of ABAP Program)
It's a global reusable technique.
With the help of includes, we can reuse same set of
statements in several programs.
we can code once in an include program and reuse that
include in the other programs wherever it is required.
Include programs cannot call/Run themselves. (i.e.,
dependent on the executable
Programs)
Include programs cannot
contain PROGRAM or REPORT Statements as a first statement.
Syntax:
INCLUDE
<name_of_the_include>.
We can create
include programs in 2 ways:
1. SE38 Transaction -> Enter the include name (Starts
with 'Y/Z')
Choose Type as
'Include Program' -> save in a package & Transport request.
Implement the logic
in Include.
and use the Include name in any executable Program.
2. We can implement directly in any executable programs as
mentioned below:
Write the include with the proper syntax in the
program.
INCLUDE <name_of_the_include>.
Example:
include ZPROG_TOP.
Double click on
Include Name (name of the Include (For Ex: ZPROG_TOP))-> press 'Yes'
->Pop-up screen will appear-> Program type will come
automatically as 'include'.
Save-> Keep it in a Package & request.
Then write your code in Include.
Requirement:
write the 4 programs for 4 arithmetic operations (Addition, Multiplication,
subtraction, Division)
Write the simple
code as below for Addition:
REPORT ZINCLUDE_EXAMPLE1.
DATA OUTPUT TYPE I.
PARAMETERS: P_INPUT1 TYPE I,
P_INPUT2 TYPE I.
OUTPUT = P_INPUT1 + P_INPUT2.
WRITE:/ 'The Addition of 2 Variables is', OUTPUT COLOR 3.
PARAMETERS: P_INPUT1 TYPE I,
P_INPUT2 TYPE I.
OUTPUT = P_INPUT1 + P_INPUT2.
WRITE:/ 'The Addition of 2 Variables is', OUTPUT COLOR 3.
Input:
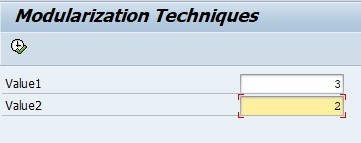
Output:
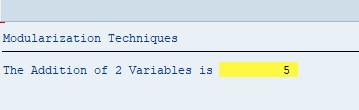
Write the above program using Include inside it:
reason to use include is w.r.to above requirement is:
As per the given requirement we need to do 4 programs for 4
different operations.
In this case declarations are same but change is there in
the calculation part.
Hence, we can reuse the declaration
part into include Program in this program
then we will use ths include in other programs.
Please follow below steps to achieve this:
REPORT ZINCLUDE_EXAMPLE1.
*& Program to do Addition of 2 Variables
INCLUDE ZCOMMON_DECLARATION. " Include for Declarations
OUTPUT = P_INPUT1 + P_INPUT2.
WRITE:/ 'The Addition of 2 Variables is', OUTPUT COLOR 3.
*& Program to do Addition of 2 Variables
INCLUDE ZCOMMON_DECLARATION. " Include for Declarations
OUTPUT = P_INPUT1 + P_INPUT2.
WRITE:/ 'The Addition of 2 Variables is', OUTPUT COLOR 3.
Save-> Check-> Activate and Observe the Output.
Double click on Include to write/see
the logic in it:
INCLUDE ZCOMMON_DECLARATION. " Include for Declarations
Logic:
Now, create a new program for subtraction and reuse above
created Include in that program as below:
Code:
REPORT ZINCLUDE_EXAMPLE2.
*& Program to do Subtraction of 2 Variables
INCLUDE ZCOMMON_DECLARATION. " Include for Declarations
OUTPUT = P_INPUT1 + P_INPUT2.
WRITE:/ 'The Subtraction of 2 Variables is', OUTPUT COLOR 2.
*& Program to do Subtraction of 2 Variables
INCLUDE ZCOMMON_DECLARATION. " Include for Declarations
OUTPUT = P_INPUT1 + P_INPUT2.
WRITE:/ 'The Subtraction of 2 Variables is', OUTPUT COLOR 2.
Save-> Check-> Activate and Observe the Output.
Note: Repeat the same steps for the other arithmetic
operations.
3.Function Modules
-Transaction code to
Create Function Groups and Function Modules is SE37 / SE80.
-It's one of the global modularization technique.-Function modules are stored in a Central Library. (i.e., Function Group)
-Function modules are having reusable logic/code
which can be used in
different programs in Abap.
-Function modules can be executed independently using 'SE37'
transaction.
Where to use the function modules?
we use function modules inside programs, other function
modules and methods.
Pre-requisites to create a Function Module
- Function group
Function Group:
-A function group is a collection of related function modules.
-Function group act as a main
program for all of its function modules.
-When we are using CALL FUNCTION statement in ABAP program,
the system loads the entire function group in with the program code at runtime.
- Each function group is identified by a four-character
identifier called a Function Group ID.
Note:
Every function module belongs to a function group.
Scenario to understand
"Function group" concept:
If you get 3 requirements related to 'Employee' like mentioned below:
Requirement 1:
Create a Function module to display the Employee name using Employee No.
Requirement 2:
Create a Function module to display the Salary details of an employee based on
Employee no. (Reuse)
Requirement 3:
Create a Function module to display the Tax details of an employee.
Solution:
to meet the requirement, we will create 3 different function
modules for above 3 requirements
For example:
Function Module 1:
ZFM_GET_EMP_NAME
Function Module 2:
ZFM_GET_EMP_SALARY
Function Module 3: ZFM_GET_EMP_TAX_DETAILS
As a pre-requisite, we need to assign Function group for every function
module.
For Above
requirement,
instead of creating multiple function groups we can create
one Function group and assign it while creating all the above function modules
(Reason is: all the above function modules are related Function modules)
For example:
Create a Function group like below and assign it in all FM's
ZFG_EMPLOYEE (Function group for all employee related logic)
Note:
Take any function module and check the tree structure of
Function group in SE80/SE37 Transaction.
Components of function group:
1. Main program.
(Name Starts with 'SAPL')
2. Top include. ----> To define Global
Data.
3. UXX include. ----> For FM which got
created.
4. Function module includes.
Steps to Create Function Group:
1.Execute Transaction SE37.
2.Choose 'GOTO' from
Menu-> Function Groups-> Create Group
3.Pop-Up Screen will appear.
In the Pop-Up Screen
Enter Function Group
Name (Starts with 'Y/Z').
Enter Short
Description.
Click on SAVE

Save it in a Proper Package
Name/If Package name not available then choose Local Object.


If you save it in a proper package name then save it in a
Transport request.

Once you click on Continue/Enter button then Function Group
will be created.
Check the below message on bottom of the screen.
Note:
Function Group status
will appear as 'Inactive' as soon as
its created.
To activate the Function Group, we need to do below
steps:
Transaction SE37-> goto-> Function Groups->Display
Function Group
Click On Continue.
-> One Screen
will appear as below -> Choose Main Program Button.
-Below is the Main Program of Function Module:
Click On the 'Display Object List' Option
-> Left side one
small screen Portion will appear as below and it shows your Function Group
-Right click on the Function Group Name and Choose Activate
-Press Enter.
That's it..!
Steps to Create Function Module:
We will take a
requirement to discuss on how to create a function module
Requirement:
Create a Function module to display the Employee Name based
on Employee No (Use Table as : PA0001)
Steps:
1. Execute Transaction: SE37
Click On Create
Pop-Up Screen will Appear as below:
Click on SAVE.
Click On Continue..It will
take us Function Module.
Enter Import(Input) Parameters
Enter export(Output) Parameters
Write the Code/Logic in Source code Tab
SAVE->Check-> Activate
Execute (F8).
Pass a Valid Input Parameter:
Click On Execute (F8)
Check the output:
Note:
We have checked the Function module independently in SE37 Transaction.
With this we have ensured that the Created Function module is working properly.
How to use Function Module in Programs
Execute Transaction SE38
Create an Executable Program
Click On Pattern->
and Click on Continue.
Pass Inputs to Function
Module.
Save-> Check->Activate
Check the Output:
4. Subroutines
·
It's a reusable technique.
·
Mostly we can use this technique for local (within
a single Program) modularization.
·
Using subroutines, we can avoid code
redundancies (reducing repetitive code) of a program
Types
of subroutines:
1. Internal
subroutine
2.External
subroutine.
1. Internal subroutine: Definition and Calling of subroutine is exists in the same
program.
Syntax:
Step1:
Write a calling statement using below statement.
Calling the Subroutines
PERFORM <Subroutine Name> USING
<Actual Parameter1> < Actual Parameter 2>
CHANGING < Changing
Parameter 1> <changing2>.
Note: Using and Changing Parameters are always not necessary while writing
subroutine.
Double click on Subroutine Name then you will get below section (Subroutine
definition):
Defining Subroutine
FORM <Name> USING
<P_INPUT1> <P_INPUT2>
CHANGING <P_ OUTPUT1>
<P_ OUTPUT2>.
ENDFORM.
Example:
Program
without using Subroutines.
*&
Program to add 2 integers repetitively
REPORT
ZSUB_ROUTINES.
DATA: var1
TYPE i,
var2 TYPE i,
res TYPE i.
var1 = 100.
var2 = 20.
res = var1
+ var2.
WRITE:/
'The addition of var1 and Var2 is', res.
var1 = 200.
var2 = 40.
res = var1
+ var2.
WRITE:/
'The addition of var1 and Var2 is', res.
var1 = 300.
var2 = 40.
res = var1
+ var2.
WRITE:/
'The addition of var1 and Var2 is', res.
Output:
Program
using Subroutines.
Above
program Using Subroutines
*&-----------
REPORT
ZSUB_ROUTINES.
DATA: var1
TYPE i,
var2 TYPE i,
res
TYPE i.
PERFORM
do_addition USING 100 20. "
Calling Statement
PERFORM
do_addition USING 200 40. "
Calling Statement
PERFORM do_addition
USING 300 40. " Calling Statement
*&---------------------------------------------------------------------*
*& Form DO_ADDITION
*&---------------------------------------------------------------------*
FORM DO_ADDITION
USING P_var1 " Definition of Subroutine
P_var2.
res =
p_var1 + p_var2.
WRITE:/
'The addition of var1 and Var2 is', res.
ENDFORM.
Output:
2.External subroutine:
are defined
in in one program and are called in a different program.
Syntax of calling the external subroutine:
PERFORM
<SUBROUTINE NAME> IN PROGRAM <PROGRAM NAME>
USING <ActParam1> <ActParam2>...
CHANGING <ChangingParam1> < Changing
Param2>...
Example: Program
1
Report ZPROGRAM1.
DATA Result TYPE I.
*& Calling Statement
PERFORM do_addition
USING 10 20
CHANGING
Result.
WRITE Result.
*& Definition of Subroutine
FORM
do_addition USING var1 TYPE I
var2 TYPE I
CHANGING result TYPE I.
result = var1 + var2.
END
FORM.
Save-Check-Activate
and Execute.
Observe
the Output:
30.
Now, write
one more Program to make use of Subroutine which was defined in above Program
Program Name: ZPROGRAM2
Report ZPROGRAM2.
DATA RESULT TYPE I.
PARAMETER: Param1 TYPE I,
Param2 TYPE I.
PERFORM
do_addition IN PROGRAM ZPROGRAM1
USING Param1 Param2
CHANGING
Result.
WRITE RESULT.
Save-Check-Activate
and Execute.
Pass
Inputs to Selection-Screen:
Param1
-> 40
Param2
-> 60
Click On
Execute.
Output:
100.
Awesome blog with lots of useful information on SAP Training in Chennai wonderful explanation on SAP module.
ReplyDeleteSAP Training Center in Chennai | Best SAP Training in Chennai | SAP Training Institute in Chennai